ASP.NET 5 & Core: Get App Settings Values by Static Method
Lets be clear, 99% of the time you should be using one of the standard configuration providers recommended by Microsoft.
Recently I found myself needing to cater to an instance from the 1% of cases where we need to write our own configuration provider. Essentially my use-case is that the official configuration providers do not work well with static methods and I needed a way to get values from appsettings.json from a static method within a static class.
Remember that we don't really want to write code that exposes the whole of our appsettings.json file. In a project that's being worked on by several people, you could quite quickly end up in a situation where everybody writes their own implementation for getting specific values from the app settings file.
We need to write a way for our static method to request a specific value from the appsettings.json file.
Appsettings.json Explained
The appsettings.json file is the default file used by ASP.NET applications to store configuration settings, It's normally added to your project automatically when you create a new ASP.NET 5 or Core project however you may need to create it manually if your created a console or similar application based on the Visual Studio templates.
The contents of appsettings.json might look like the below:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"DefaultConnection": "server=localhost;database=;uid=db;pwd=",
"LoggingConnection": "server=localhost;database=;uid=db;pwd="
},
"ApplicationSettings": {
"TestArea": {
"TestKey": "TestValue"
}
}
}
Our static method is going to grab a key stored under ApplicationSettings and inside an area like TestArea > TestKey.
The Code
I will now give you the code and provide an explanation below. Please make sure that you fully understand the code before pasting it into your project. The explanation & screenshots are based on the steps you would take in Visual Studio 2019 since this is most helpful for new developers, therefore some steps may differ if you're using a different IDE or text editor.
Go ahead and begin by installing the following Nuget packages.
Microsoft.Extensions.Configuration
Microsoft.Extensions.Configuration.Binder
Microsoft.Extensions.Configuration.Json
Microsoft.Extensions.Configuration.FileExtensions
You can install a Nuget package by right clicking on the project and selecting Manage Nuget Packages.
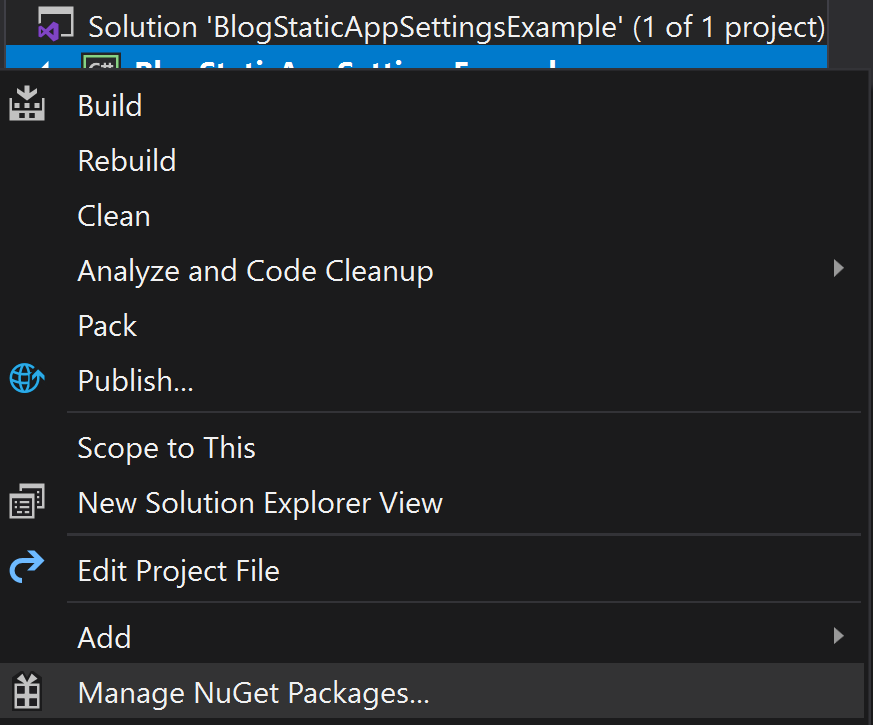
Or alternatively in the command line by typing the following:
Install-Package Microsoft.Extensions.Configuration
Install-Package Microsoft.Extensions.Configuration.Binder
Install-Package Microsoft.Extensions.Configuration.Json
Install-Package Microsoft.Extensions.Configuration.FileExtensions
Now create a file called SettingsConfigHelper.cs by right clicking on the project and selecting Add > New Item > Class File and name it SettingsConfigHelper.
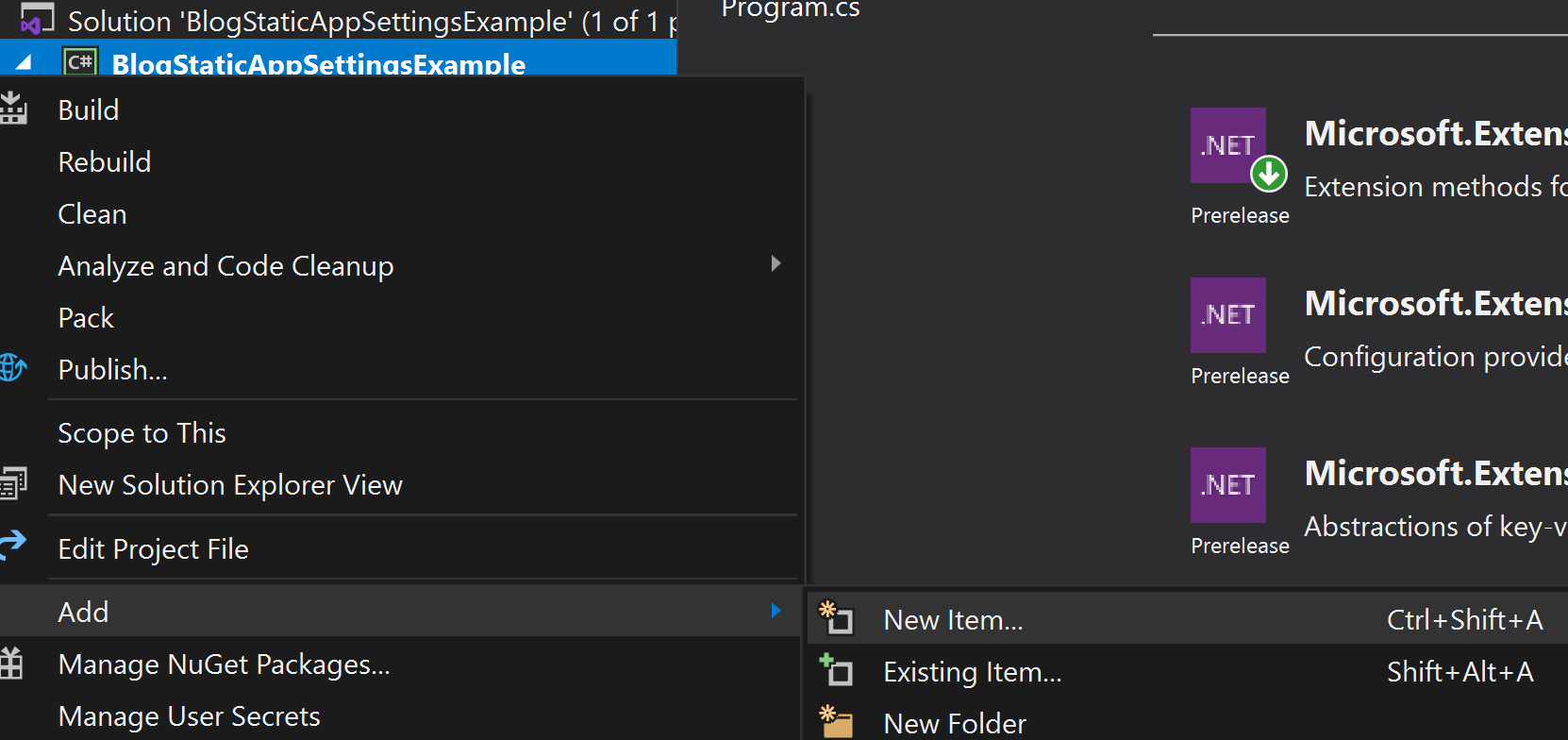
Paste the following code into your newly created file.
using Microsoft.Extensions.Configuration;
public class SettingsConfigHelper
{
private static SettingsConfigHelper _appSettings;
public string appSettingValue { get; set; }
public static string AppSetting(string Key)
{
_appSettings = GetCurrentSettings(Key);
return _appSettings.appSettingValue;
}
public SettingsConfigHelper(IConfiguration config, string Key)
{
this.appSettingValue = config.GetValue<string>(Key);
}
// Get a valued stored in the appsettings.
// Pass in a key like TestArea:TestKey to get TestValue
public static SettingsConfigHelper GetCurrentSettings(string Key)
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddEnvironmentVariables();
IConfigurationRoot configuration = builder.Build();
var settings = new SettingsConfigHelper(configuration.GetSection("ApplicationSettings"), Key);
return settings;
}
}
We're now going to create another file called Settings.cs. Paste the following code in.
public class AppSettings
{
public string GetTestArea()
{
return SettingsConfigHelper.AppSetting("TestArea:TestKey");
}
}
As you add more configuration data to the appsettings.json file, you will place additional methods that call the new specific values from the specific keys that you are looking for. Like what we see in the method called GetTestArea. The most modular approach is to have one method responsible for getting each individual value.
In my opinion, its best to split the getter methods from the file containing the code that handles the configuration builder, purely from a clean code standpoint. However you can always merge them into one file if you wish.
I hope this guide has been useful. Reach out to me on Twitter if you have any questions.