Log Errors & Events to Sumologic in C# using HTTP
Sumologic's cloud logging service is a great way to push log files and events into the cloud. I've used it myself for keeping track of error logs and flagging issues on test environments.
Without Sumologic logging I'd need to dial into the server and manually pull the log every time I wanted to investigate an issue. This is extremely time consuming and doesn’t even take into account the fact that Sumologic also offers alerts, metrics & visualisations. Sumologic is also great for serverless solutions that would otherwise be difficult to setup logging due to the fact that many solutions like AWS Lambda do not have permanent storage.
I have an application written in C# that needs to stream logs into Sumologic via HTTP requests. Luckily Sumologic have a fairly straightforward process for setting up the sending of logs via HTTP, and we can very easily write some C# code to push the logs to Sumologic's endpoint.
Batching & Other Considerations
If you read the documentation for setting up a HTTP source, you'll notice that they prefer it if you don't open more connections than necessary and you should look to batch logs together.
HTTP POST requests should not be more than 1mb in size. These considerations are likely in place to limit the network resources needed for the transfer.
Setting Up The Endpoint
Before you start recording data to Sumologic, you first need to setup a HTTP endpoint. Start by clicking on the Setup Wizard button from the online portal.
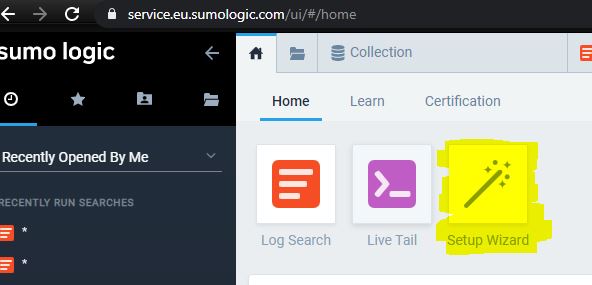
Then select the option to Set Up Streaming Data. You'll see lots of options for different types of logs, here we just want to select Your Custom App.
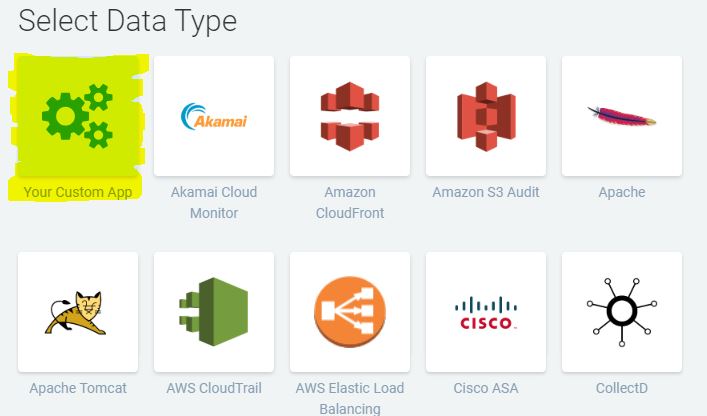
Now choose the option for HTTP Source.

Choose a custom category for the data. The source category is your own custom metadata type and can be anything you want it to be. The standard pattern usually looks something like "<env>/<servertype>/<app>/<logtype>". You can also select a time zone at this step, I usually set all my log times to UTC for consistency. When you're ready, click Continue.
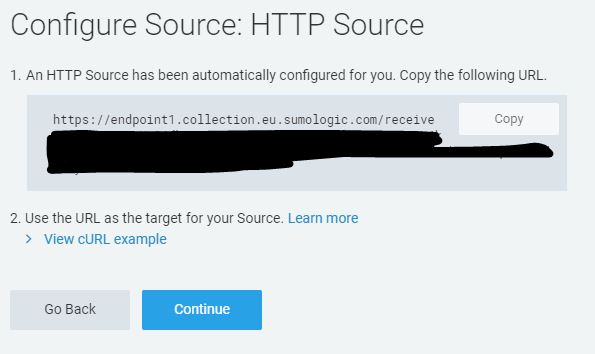
A new URL will be generated for you. Keep this secret because anyone can post to this URL and it will consume your quota.
The Code
Here is the code used to post logs to Sumologic.
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace LogicShared
{
public static class LoggingSumoLogic
{
private const string URL = "INSERT YOUR SUMOLOGIC URL";
private async static Task<string> PostSumoLogicLog(string URL, string dataToLog)
{
var httpContent = new StringContent(dataToLog, Encoding.UTF8, "application/json");
var client = new HttpClient();
var response = await client.PostAsync(url, httpContent);
return response.StatusCode.ToString();
}
}
}
Before this will work, you need to replace the URL variable with your own endpoint and because this is an async method, you need to call it asynchronously. Also remember that a statuscode will be returned, so you can use this to check if the request was successful.
The dataToLog parameter must contain the string of data that you want to log. As you can see, the method builds a HttpClient that posts our data with UTF8 encoding to the URL we have specified before the result is returned. It's as simple as that!